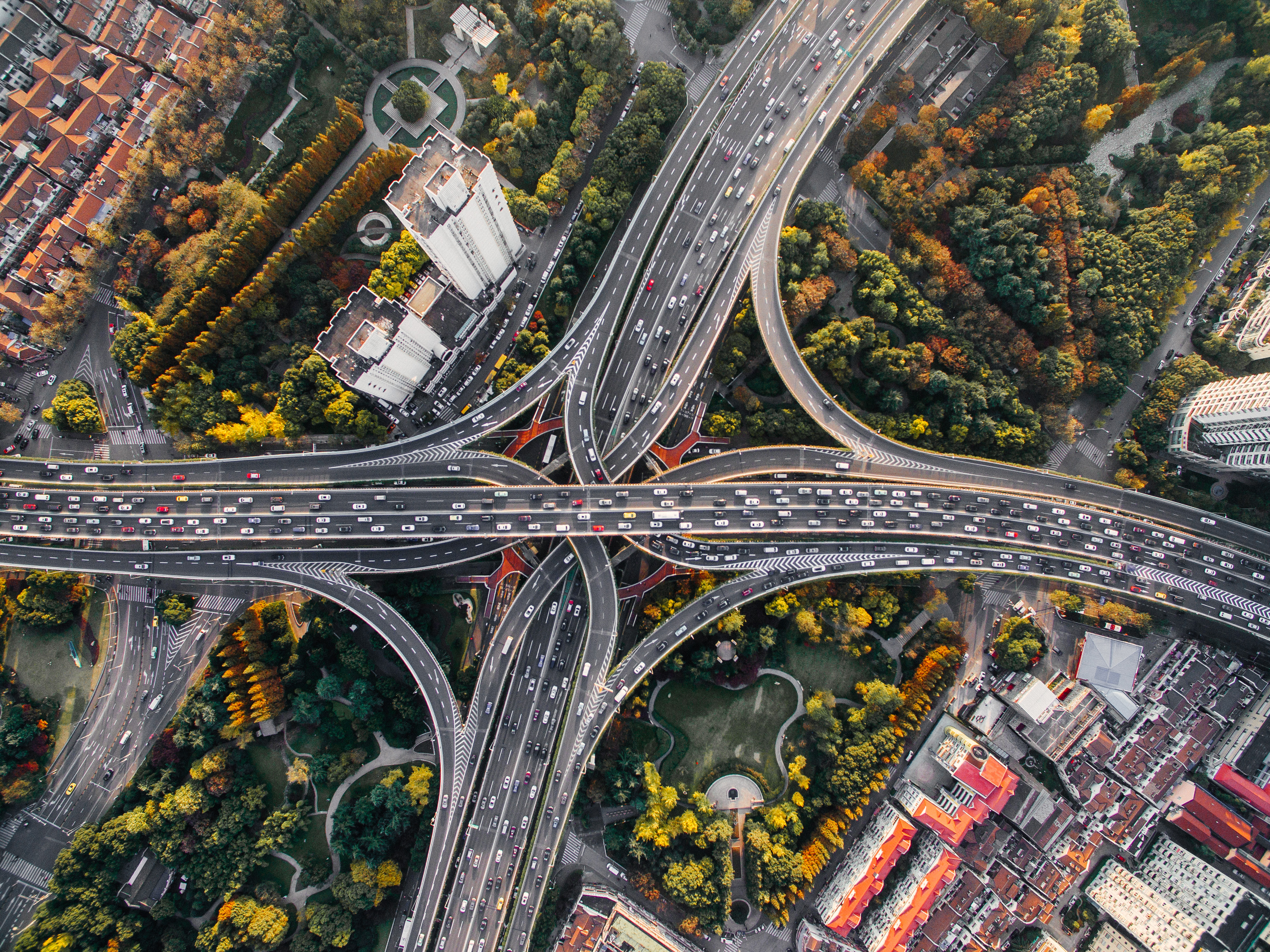
Fetching and displaying data with React Query and AG Grid
React Query offers a declarative approach for fetching data with hooks. Here's how you can combine it with AG Grid to create sleek data grids.
Why React Query for Data Fetching?
"People are really much more interested in data fetching and caching than they are managing state." - Mark Erikson, Redux Maintainer
The first question we should naturally be concerned with when facing any new technology is: "Why is this better than the existing alternative?" Luckily in our case, the benefits of using React Query's useQuery hook over useEffect are rather clear. useQuery offers a much more declarative and natural API for fetching data than useEffect, which is a rather manual way of doing data fetching in React; so much so that the React team themself no longer recommends using useEffect for data fetching.
Normally when you use AG Grid and other data tables with React, you must keep all of the data for your data table in the state of your components. Thus any kind of updating, caching, etc. of the data must be handled manually by the developer through useEffect and other means. React Query offers a much cleaner approach to fetching and managing data for data tables than useState and useEffect alone provide.
Creating a Data Table with React Query
This tutorial will focus on building a data table of my (fictional) guitars using React Query and AG Grid. For more deeply customizable grids, I would recommend using React Table, however AG Grid offers a robust and simple out of the box solution that is great for the purposes of this demonstration.
Getting Started
The first step is to write a function to get the data from the backend. This tutorial won't cover creating the backend, but I've set up a simple server that returns a JSON response from the guitars endpoint. We use fetch to write a function that returns this data like so:
1// src/GuitarTable/queryFunctions.tsx
2export async function getGuitars() {
3 const response = await fetch("http://localhost:8000/guitars");
4 const data = await response.json();
5 return data;
6}
Writing the Query
useQuery takes three arguments: A query key, the query function, and the query config. For this query, we don't need any non-default config, so we do not need to pass the third argument. Here is how we can use the above function within useQuery to synchronize our front end with the server data:
1// src/GuitarTable/queries.ts
2import { useQuery } from "react-query";
3import { getGuitars } from "./queryFunctions";
4export const useGuitars = () => {
5 return useQuery("guitars", getGuitars);
6};
While I have stylistically chosen to wrap this in a custom hook, you can also just put the useQuery directly in your component.
Creating the Table
To create the AG Grid table, all we really need to do is define the data grid's columns, i.e. the AG Grid columnDefs.
1//src/GuitarTable/columnDefs.ts
2export const columnDefs = [
3 { headerName: "Brand", field: "brand" },
4 { headerName: "Model", field: "model" },
5 { headerName: "Price", field: "price" },
6 { headerName: "Year", field: "year" },
7 { headerName: "Color", field: "color" },
8 { headerName: "Type", field: "type" },
9 { headerName: "Country", field: "country" },
10];
11export const defaultColumnDef = {
12 width: 150,
13 flex: 1,
14 sortable: true,
15 filter: true,
16 floatingFilter: true,
17};
Using the Data in the Table
Now that we have written the useQuery and wrapped it in a custom hook, we simply need to hook up our query to the AG Grid React component, like so:
1// src/GuitarTable/GuitarTable.tsx
2import "ag-grid-community/styles//ag-grid.css";
3import "ag-grid-community/styles//ag-theme-alpine.css";
4import { AgGridReact } from "ag-grid-react";
5import { columnDefs } from "./columnDefs";
6import { useGuitars } from "./queries";
7const GuitarTable = () => {
8 const { data } = useGuitars();
9 return (
10 <div>
11 <h1>Max's Guitars</h1>
12 <div
13 className="ag-theme-alpine"
14 style={{
15 height: "500px",
16 width: "600px",
17 }}
18 >
19 <AgGridReact columnDefs={columnDefs} rowData={data} />
20 </div>
21 </div>
22 );
23};
24export default GuitarTable;
25
And just like that, we've built a robust, fast, sleek data grid. There is of course a lot more to both React Query and AG Grid, and I plan to make a second part to this tutorial soon to go into more detail. The full code for this project can be viewed and cloned from the Github Repository here. I've also deployed a live demo.